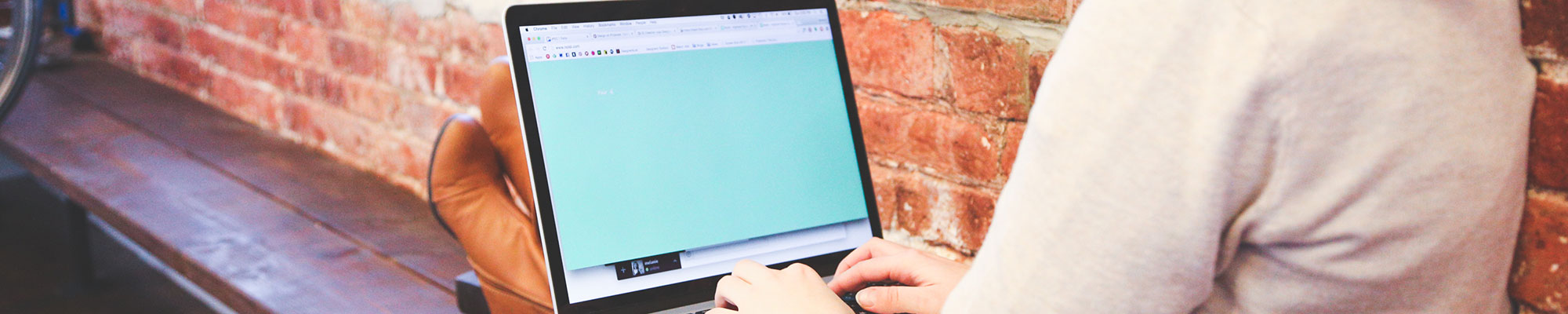
JavaScript: Intro to Programming
What is it?
JavaScript is a scripting language commonly implemented as part of a web browser in order to create enhanced user interfaces and dynamic websites. It's a good language for beginners, because it allows you to quickly implement interactive techniques in HTML pages. The basic features you learn with JavaScript will be able to be applied to other languages.
Why should I learn it?
JavaScript adds the elements of logic-based programming to a website. You'll be able to interact with users and make sites that are responsive to users' inputs.
What can I do with it?
You can change the elements of a page based on a user's input. You will learn about manipulating the Document Object Model (DOM) to customize pages and present data.
How long will it take?
Programming is something that you learn over time, through practice. But in a few hours, you will be introduced to the basic elements of programming that you will be able to apply to your own projects. Subsequent exercises will provide examples of programming concepts and how you can integrate them into Web projects.
History of JavaScript
JavaScript is a scripting language commonly implemented as part of a web browser in order to create enhanced user interfaces and dynamic websites. There are a few things to understand about JavaScript before we get started.
JavaScript started in the mid-90s to provide a lightweight language to add interactivity to Web experiences, as opposed to Java, which was positioned as a language for developing enterprise systems. JavaScript was designed to be easy to implement for designers and beginners.
- Although they are rooted in similar syntax, JavaScript is not the same as Java. Java is not short for JavaScript. While the names sound similar, they are completely different programming languages.
- JavaScript can be run client side (on the user's computer) or server-side (executed on a Web server. Node.js is a server-side JavaScript environment).
- JavaScript is the foundation for code libraries like JQuery. It is the basis for interactive development libraries like React.
JavaScript has gone through many iterations since its inception. These iterations are standardized by the European Computer Manufacturers Association (ECMA). You will often see JavaScript versions referenced as ECMAScript. A major update to JavaScript was made with ECMAScript 6 or 2015, and we discuss many of those features -- including let and const bindings, template strings, default properties for functions, arrow functions and array features -- in these tutorials. Learn more about the History of JavaScript.
Getting Started
The following exercises will introduce you to a range of concepts using JavaScript. But these are common concepts used across many different programming languages that you will be able to apply as you continue to learn. Programming is really a matter of creativity and problem-solving, so you just need to understand these basic elements to get started practicing the thought processes involved with solving problems. You solve technical problems by developing a series of steps, the algorithm, that will be executed by the computer.
For the first series of exercises, we’ll be practicing in Chrome’s console. This is an environment that let’s you run code and see the result. Later, we will be implementing JavaScript in HTML pages.
In Chrome, choose View, Developer, JavaScript Console. Or ctrl-click on the screen and choose Inspect. You'll be able to copy and paste the code from the code samples below into the console. You can find more information about Chrome Developer Tools.
Basic JavaScript Syntax
Keep these basic syntax rules in mind as you begin coding:
- End lines with semicolon ;
- Put arguments/parameters in parentheses ( )
- Period between object and method or property
- Strings in quotation marks
- Opened elements must be closed, so pay attention to quotation marks, parentheses and curly braces
These syntactical rules may not make sense right now, but will become clearer as we work through the exercises.
Your First Program
Let's jump in. We'll start by writing the string "Hello World!" to the console and screen. Use both of the methods to the right in the console. Don’t worry if you see "undefined" in the console after the code returns. The console is attempting to determine the data type that the code returns, and in most cases in our exercises that is "undefined."
Take a look at the structure of the code below. "console" is the object and "log" is the method. You separate them with a period (.). Basically you are asking the "console" to perform the action "log". The content within the parentheses is the "argument" of the method or function.
When using the Chrome console, you don't need to use console.log. It is optional. If you just type your test or commands into the console, it will execute as console.log. Later we will use other methods to write to an HTML document.
Note: this tutorial no longer uses the document.write command that is demonstrated in some of the videos. We will demonstrate concepts for writing to the window in an html file in the next lesson. The console.log function demonstrates each concept in this tutorial.
Code Sample - First Program
"Hello World" is a programming tradition. When starting any new language, learning how to write the string "Hello World" is typically the first lesson. But you can choose any string you want to log to the console.
Data Types
There a are number of different data types - String, Number, Boolean, Array, Object, Null. Programming languages have syntax and functions that work with a variety of data types.
We will mainly be concerned with strings and numbers, later adding booleans, arrays and objects - each is described below.
Strings
Strings are simply text. They are represented in quotation marks.
Length
Length is a string property that returns the length of the string. Sometimes a program needs to count the number of characters in a string. Perhaps you are trying to validate a password that needs to be 8 characters or more or determine if the number of letters in a tweet is less than 140.
Code Sample - Length
Use either of the methods below to calculate length of a string.
This returns a number that is the length of that string, including the space. So in the example above, the length returned should be 12. It includes the exclamation point.
Concatenation
Use concatenation to join strings and/or variables. The empty space between the quotation marks (" ") represents a space. The plus sign is the concatenation operator. If you capture multiple strings or variables that you need to repeat together, you use concatenation. For example, if you have collected first and last names from users, you might want to return them together in a sentence. You might want to include a standard welcome message with the person's name.
Code Sample - Concatenation
The console should return Hello Cindy!
Variables
Use variables to store numbers and strings. When you collect information from a user on a form, you will store it in a variable. Then you can use it when you need to in a program. You can use variables to store strings, numbers and other data types. The var prefix is optional. We will be using generic variables in these tutorials, but there is also the "let" prefix for assigning block scope to a variable and "const" for defining constants whose values should not change throughout a program.
Another method for joining strings and variables is to use template literals. Template literals allow you to identify a variable as such, ${variablename}, and use it in a string that is identified with a backtick ` (the item to the left of the number 1 on your keyboard). This allows you to present the variables within the lines of text. This will be helpful as you start writing html with variables.
You can use the typeof method to determine the data type that JavaScript is interpreting of a variable.
typeof variablename;
Code Sample - Variables
Use variables with concatenation to make a welcome message. Try both examples below.
Try the above examples using your own name in the variables. Did the console greet you properly? Notice in the 2nd example that instead of concatenating a space " ", we simply added a space to the "Hello " string. In the third example, we simply integrated the template literals that represent the variables within the string.
Substrings
A substring identifies a portion of a string. Use these scripts to find your popstar name (like JLo). Consider the algorithm: find the first character of first name and the first two characters of last name. What's your popstar name?
The substring method takes two arguments - a start and end position in the string, the first position in the string is 0.
Code Sample - Substrings
Notice that we used a combination of variables, the substring method and concatenation in two different ways to achieve the same result. Often in programming, there are multiple ways to solve a problem.
Exercise: Write your own program that takes first and last name of the user and creates a username with the first five characters of firstname and first three characters of last name. Test in many different scenarios. What happens when there aren't enough characters provided?
Numbers
You can use numbers for a variety of operations in JavaScript. Numbers can be integers (whole numbers) or floats (decimals). You can use numbers to make calculations. They can be stored in variables to be used throughout a program. The code samples to the right show examples of using numbers for addition, subtraction, multiplication and division. The final example calculates the modulo or remainder of division calculation.
Code Sample - Numbers
Try each of the following in the console.
You should see the correct answers for the different operators for math functions.
Alerts, Confirms and Prompts
You use alerts, confirms and prompts to gain the attention of users via a dialog box.
Alert - a general warning
Confirm - answer yes or no before you can continue.
Prompt - answer anything - creates a variable. This is our first example of being able to interact with a user and store their input.
Code Sample - Alerts, Confirms, Prompts
Try the following commands individually in the console.
The prompt will store the result in variable so it can be used again in the program. We'll do some exercises in the next lesson using prompts, but then we'll move on to using form elements for our interactivity.
if Statements
Perhaps the most important aspect of programming is being able to apply logic to decision making. When given a choice between multiple options, you need to be able to provide instructions for each scenario. That's where "if statements" can be used.
A boolean is a special kind of data type. You use booleans to test if something is true or false. You can combine with an if/else statement to define different outcomes. Notice that the if statement actions are delineated with curly braces. You can also test for mathematical statements like greater than >, less than < or equal to ===. You can also use !== to test if something is not equal.
Comparisons (= or == or ===)
A single equal sign (=) indicates assignment, usually used to assign a value to a variable. If you are doing a test for comparison, then you use 2 equal signs (==) or 3 equal signs (===). Three equal signs designate "strict equivalence" which eliminates some of JavaScripts loose interpretations of numbers and strings. We used the assignment and comparison operators in the examples above.
You can use operators to include statements to indicate "and" and "or" in your booleans. These operators are && and ||.
Examples:
2+2===4; This would evaluate to "true"
name="Cindy"; This is example of assignment
if(name.length >=3 && name.length <= 10) {...} This is example of comparison in an if statement, using the && operator. This tests the condition that the length of the variable is between 3 and 10, thus creating a range.
You can use "if else" to include additional conditions for the if statement. If you are working on a program that requires several "if else" statements, you might consider using the switch syntax instead. We won't go over it, but review that example at the link, to familiarize yourself with its usage.
Code Sample - if Statement
Try changing the name to a word with a different number of letters, i.e. name = "Catherine" and see what you get. Simply rerun the script and change the word in the "name" variable.
Exercise: Write a script that looks at a password and evaluates if it is greater than or equal to 8 characters. See if you can use a prompt to input the password you are testing.
Console Tips: You can use the up arrow key to scroll back through code you have used before in the Console. And a Shift-Click lets you add a line to your code without submitting it in the Console. Use these techniques to modify code you have already created.
Loops
Loops allow for iteration through a cycle. In programming, you often need to perform an action over and over again, until a certain condition is satisfied. There are two kinds of loops: while and for.
The "while" loop is a series of statements that define initializer, condition and iterator to describe how the loop should operate.initializer - where you start counting; often 0 or 1, but can be any number that you want to start the loop's counting. You store the initializer in a variable, often used with "i".
condition - this is the condition that must be satisfied for the loop to end
iterator - this is the instruction for how to increment the loop. Loops usually increment by 1 during each cycle, but can increment by an amount.
The examples below do the same thing, incrementing the iterator by one. You include this at the end of the loop so it executes each time until the condition is met.
You provide the instructions to be executed during each iteration of the loop within the curly braces.
The "for" loop reduces some of the coding by having three statements in the parentheses, separated by a semicolon (;) - an initializer, a condition to test and an iterator. See the example to the right. The iterator can also be used in the loop to return the place each time through the loop. But be familiar with the while loop syntax, because there are some problems where it may need to be used in lieu of a "for" loop, particularly when some of the elements are unknown when the program initializes.
Code Sample - Loops
Code Sample - For Loop
Or if you want to get fancy, you can add the iterator variable to the write statement to have a different number in each line.
Exercise: In the console, write a for loop that prints out the numbers from 1-10.
Then modify the script so that it prints out double the numbers.
Now add an if statement to only write the doubled values if i is greater than 5.
Finally, how would you write the original script to only write the even numbers from 1-10? Try writing that program.
Arrays
Arrays allow you to store a collection of information in a variable and then access it via its index number. So far, we have stored one item in a variable. Arrays let you store a list of items that can be accessed by an index number. Index numbers start with 0. Enclose elements of an array in square brackets.
Arrays are useful for storing information collected from each iteration of a loop. They can also be used to store simple data in a text format.
You initialize an array like a variable, but you include the list items with square brackets.
favGroups = ["Old 97s", "Spoon", "Wilco"];
You can add an element to an array with the push method.
favGroups.push("Quiet Company")
The favGroups array would now have four elements in it after pushing the new item. The new item is added to the end of the array.
Code Sample - Arrays
This accesses the item in the array in location 1. Notice that it isn’t the first item in the array. Arrays start with 0, so to access the first item use favGroups[0].
You can use a while or for loop to iterate through an array, but we will be using for loops from now on. Concatenate a break so the items appear on a different line. Your condition uses the length property of an array to determine how many items are in it.
Objects
Objects are similar to arrays except they store items in key-value pairs rather than accessing them by their index number. This allows us to store more of a dictionary of data, including an item and it's meaning. Instead of using square brackets, you use curly braces for objects.
Code Sample - Objects
We can use an array in conjunction with an object to collect multiple objects. In this example, the variable "bands" is an array that holds multiple objects, each band's name, city and genre.
The example above finds the object at position [1]in the "bands" array.
And we can loop through an array of objects just like we did with a simple array. Your condition uses the length property of the array to determine how many items are in it.
Functions
Functions allow you to define an operation and then use it later. Notice the statements in the function are enclosed in curly braces. Then we call the function using its name to execute it. Functions can be stated or developed in a variable, depending on how you want to use the result later.
EMCAScript 6 introduced arrow function syntax that simplifies function definition. See the examples. These exercises generally use the "function" keyword to specifically highlight its usage, but you may see scripts that use the arrow notation as you learn more about JavaScript.
Parameters and Arguments
Often you will need to pass something into a function, a number or string or a variable calculated from elsewhere in the program. This is called a parameter. When you call the function, you provide the argument that replaces the parameter in the function. Now you can call this function multiple times with different inputs.
EMCAScript 6 introduced the inclusion of default values for parameters. This allows the function to work, even if no values are passed into it when the function is called. See the examples.
Code Sample - Functions
Write the function below that prompts for a name and provides a greeting. Then call it with the function name.
Code Sample - Parameters and Arguments
Exercise: Try to write a function that takes two parameters (you separate the parameters with commas in the function declaration) and then multiplies two numbers when passed to the function when it is run. The code below will get you started. Modify it to also add the numbers and concatenate some text to identify both the product and the sum in the answer.
You can pass any two numbers into it to have it answer with the product. Think about what you need to do to get the sum to work. This is the beginning of programming a calculator!
Comments
You may have noticed comments in some of the code examples. Developers use comments to leave notes in the code, provide instructions for other developers or to provide clues to certain techniques. It helps to organize code. Comments can also be used to temporarily remove and test code without having to delete it.
// This is a comment
/* This is a multi-line
comment */
Note: basic html comments are handled by <!-- -->. We'll see more of this as we start writing JavaScript within HTML pages.
Moving On
Now you have a basic understanding of programming concepts. In the next exercise, you will go through some exercises that will show you how to implement these techniques in HTML pages.