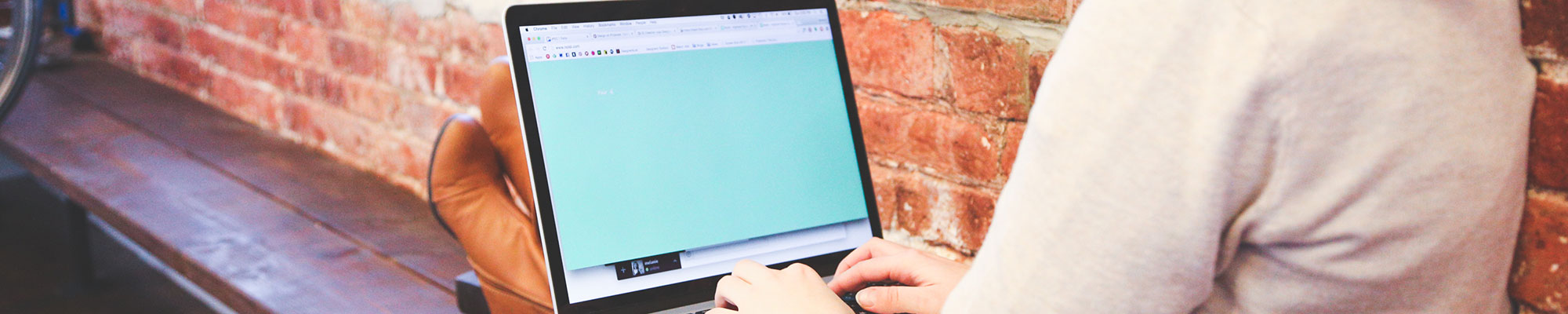
JavaScript Frameworks - Vue.js
What is it?
JavaScript frameworks and libraries provide the structure for making web apps in the JavaScript environment. There are many different types of JavaScript frameworks/libraries, but learning the basics of one will help you understand the ecosystem.
Why should I learn it?
The web development environment now includes these frameworks and libraries. It is important to understand the role they play in creating interactive web applications.
What can I do with it?
You can create interfaces and interactives that run on the web. You can take advantage of templating features that prevent duplication of effort.
How long will it take?
Learning advanced web development is a lengthy process. These tutorials will take several weeks to go through to explain the basic functionality available for interactive web development. But with time and practice, you can master the skills necessary to create functional applications.
Resources
- Glitch examples for you to Remix
- LinkedIn Learning - Learning Vue.js
- Vue.js Website
JavaScript Frameworks - Vue.js
There are several JavaScript frameworks/libraries that help you get started making a web application. They provide pre-written, standardized JavaScript code and other tools that developers use to simplify routine tasks and create interactivity on the web. We will be using Vue, but here are a few that are commonly used:
- Vue.js- JavaScript framework that helps developers build user interfaces for web applications and websites. It's designed to be simple, flexible, and easy to integrate with other libraries and projects. It was developed by Evan You, formerly of Google. It has been available since 2014. The current version is Vue3, and we will be using that version for these exercises. Be aware that future versions may change syntax associated with these exercises.
- React - open-source front-end JavaScript library for building user interfaces based on components. Available since 2011, it originated at Facebook and is maintained by Meta and a community of developers. Sites like Paypal, Netflix, AirBnB and Facebook have been developed using React.
- Other JS Frameworks include Angular, Svelte and Node.js.
- Many implementations of frameworks/libraries require the use of Node Package Managers (npm) to install the framework on your computer. But some basic functionality is able to be achieved using cloud-based links. This is the method we will use in these tutorials.
Using Vue.js
You can install the Vue framework by including links available in the cloud (cdn). You can also set up an environment on your computer with something like a Node Package Manager via Terminal for more advanced applications. For these tutorials, meant to demonstrate basic funcationlty and get an application up and running quickly, we will be using a link to the required framework in the cloud. Also, the examples we will be working on won't be those in which a database or user login is required.
Follow along with the video and use the code samples, and you will be able to create a To Do List application.
Start with a standard HTML page that has html, head and body tags configured. Include cdn links to the Vue framework in the head of the document. We are starting with a simple ToDo list example, so include a title of "Vue ToDo List".
Simple To Do List HTML
Making a To Do list might seem simple, but it is a good example of basic CRUD functionality - create, read, update, delete. We can see the way that these interactive features are easily implemented in a Vue project. This provides DOM (document object model) manipulation where inputs from users can change the view on the page.
Use the html code sample and include in the body of your page. This includes the form html for a text input for item, a dropdown for the priority and a button, but you can use any elements to capture data in your app. The v-model is the identifier used by vue to get the value from the form element. The second div is the area in which the item and priority will be presented on the page. It operates as a for loop by referencing the v-for attribute.
Within the body include the html that creates the interface.
Add Script
Now include the script that will execute the commands. It creates the app, sets up and initializes the variables in the return function and provides the addItem method. This pushes each item and its priority into an items array and mounts the app as referenced by the div id #app. Note that in the html, there reference to item.name and item.priority within the double curly braces. The script creates those items in the items array and they are referenced in the v-for loop executed in the html. The newItemName and newItemPriority are initialized after each submission of the form.
Your app should look like this after you submit a few sample items.
Remaining in the body, include this script element below the html you created.
Additional Functionality
To improve the ToDo List functionality, we need to add the features to toggle an item as complete and to delete it. First add the html for the two buttons to execute those functions. Then modify the "methods" area in the script with the sample code. The toggleComplete function finds the current item and toggles the state of being completed or not completed. There is a class binding that applies the .todo-item.completed class. By adding some CSS, we can define what that looks like. The deleteItem function removes (splice) the item from the items array.
Your app should look like this after you submit a few sample items and try the Completed (X) feature. Also test the Delete feature.
Add the button elements within the html v-for statement to be presented after the item and priority. The @click command executes the functions we will create below. Later we can format the way this looks and classes are provided for you to be able to style those elements as needed. Note the modification to the v-for loop statement to bind class.
Then in the script area, modify the "methods" to add the toggleComplete and deleteItem functions below the complete addItem statement. Look for the }, after the this.newItemPriority statement.
Then add a style section in the head and include the following to describe what a completed item should look like. This references the class binding in the v-for statement above.
Additional Formatting
There are many things we could do to better format this page. Obviously you can include styles and even Bootstrap classes to create your interface, navigation and layout grid. But for this simple exercise, let's just convert the results to be presented in a table. We create a table that has a thead area to include the headings and then the tobody executes the loop that repeats a row (tr) with a td for each element (item, priority, complete, delete).
Your app should look like this after changing the format to a table. Of course, you can use any formatting methods you want to achieve your desired layout. Pay attention to where the variables and buttons are used in the code and place them in appropriate html elements.
The app created in this manner is not something that would be useful to the general public. If you refresh the page or return to the app later, the To Do list items are erased and you have to start over. To allow the data to persist, you would have to create an application that holds the state of the data. That is beyond the scope of this tutorial, because it would require a state management library and the ability to write to a js file. But this tutorial gives you a general idea of how the basic functionality of an interactive application is achieved.
Instead of having the div that includes v-for Vue attribute, we will use table html.
Include the following css in the style area to format the table with desired alignment, borders and spacing. Of course, you could do a lot more, but this demonstrates how to use what you already know about HTML and CSS to modify this interactive application. Since we only have one table on this page, using the styles below will work, but you can also create classes and apply styles to them to specify this table on a more complex page.
Moving On
Great job! You have learned how to use Vue.js to create an interactive web app with List and Detail Views that incorporates text, images and filtering, and pulls from a dataset. Consider how you will use these techniques for other projects.